Bash Cookbook
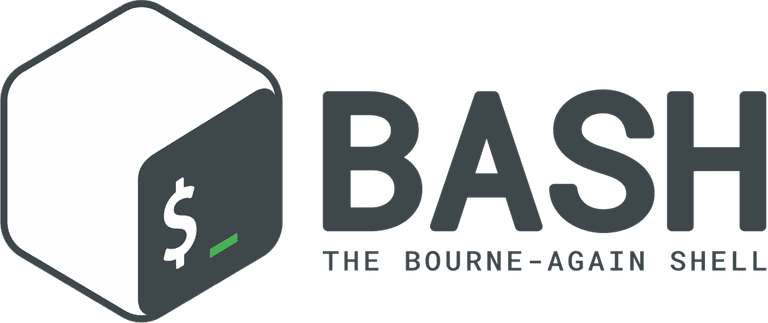
Recipes for Bash
(Bourne Again shell).
Hands-on Bash examples and guides for daily work.
Installation and Update
Linux
Bash is the default shell for Linux.
Ubuntu, Debian
apt install bash bash-completion
Red Hat (RHEL, CentOS, Fedora)
dnf install -y bash bash-completion
or
yum install -y bash bash-completion
macOS
Homebrew
brew install bash bash-completion@2
chsh -s /usr/local/bin/bash
Builitin
Basic
cd [~|-|..|<dir>]
pwd # $PWD
ls [-l|-a|-r|-h|-R]
less <file>
ln -s[f] <src> <symlink>
cp|mv [-r|-u] <src ...> <dst>
rm [-r|-f] <file ...>|<dir>
mkdir [-p] [-m <mode>] <dir>
rmdir [-p] <empty-dir>
wc -l <file>
head|tail -n <num> <file>
tail -f <file>
cut -d -f
type
$ type type
type is a shell builtin
$ type cp
cp is /usr/bin/cp
$ type ls
ls is aliased to 'ls --color=auto'
Help
help <builtin-cmd>
<cmd> --help
man|info [section] <cmd>
which [-a] <cmd>
Key Bindings
Tab
- Command CompletionCtrl+D
-exit
or EOFCtrl+C
- SIGTERMCtrl+A
/Ctrl+E
- Go to Beginning / End of Command LineCtrl+U
/Ctrl+K
- Cut from Current Position to Beginning / End of Command LineCtrl+Left
/Ctrl+Right
- Go to Previous / Next WordCtrl+Y
- PasteCtrl+L
-clear
Ctrl+R
- History Search,Ctrl+C
to QuitCtrl+Z
- SIGSTPCtrl+-
- Undo
Output
echo [-n|-e] <str>
Environment Variables
echo $PWD # current directory
echo $USER # current user name
echo $HOME # home directory of current user
Input / Output
Input
From stdin
DEFAULT="default"
read -t 5 -p "Prompt [$DEFAULT]: " VAR
if [[ $? != 0 ]]; then
echo "xxx"
fi
VAR=${VAR:-$DEFAULT}
From File
cat > /etc/pip.conf <<EOF
[global]
index-url = https://pypi.douban.com/simple
[list]
format = columns
EOF
Output Redirection
Redirect stderr
ls -l /bin/usr 2> ls-error.log
Redirect stdout and stderr
ls -l /bin/usr &> ls.log # Bash 4.0+
ls -l /bin/usr > ls.log 2>&1
Redirect stdout and stderr
ls -l /bin/usr &> ls.log # Bash 4.0+
ls -l /bin/usr > ls.log 2>&1
Sometimes “silence is golden”
ls -l /bin/usr > /dev/null 2>ls.log
Expansion
- pathname expansion:
*.txt
- tilde expansion:
~
- brace expansion:
{a,b}
,{A..C}
- command substitution:
$(echo a)
- arithmetic expansion:
$((1+1))
- parameter expansion:
$USER
$ echo text ~/*.txt {a,b} {A..C} $(echo a) $((1+1)) $USER
text /home/ly/a.txt a b A B C a 2 ly
$ echo "text ~/*.txt {a,b} {A..C} $(echo a) $((1+1)) $USER"
text ~/*.txt {a,b} {A..C} a 2 ly
$ echo 'text ~/*.txt {a,b} {A..C} $(echo a) $((1+1)) $USER'
text ~/*.txt {a,b} {A..C} $(echo a) $((1+1)) $USER
Text Processing
Subtitute
str="a-b-c"
echo ${str/-/_}_d # a_b-c_d
echo ${str//-/_}_d # a_b_c_d
Substring
str="abcdef"
echo ${str:1} # a
echo ${str:2:2} # bc
Testing Operator
-z empty
-n NOT empty
Regular Expression
*
- Any numbers of characters?
- Any single character[abc]
- Any character in set ofabc
[!abc]
- Any character NOT in set ofabc
[:alpha:]
- Any letter[:lower:]
- Any lower-case letter[:upper:]
- Any upper-case letter[:digit:]
- Any digit[:alnum:]
-[[:alpha:][:digit:]]
[:word:]
-[[:alnum:]_]*
[:blank:]
-Space
+Tab
^abc
- Any line started withabc
abc$
- Any line ended withabc
+
- More than once{n}
,{n,m}
- From (n) to (m) times
Startup Files
Login Shell Sessions
~/.bash_profile
~/.bash_login
~/.profile
/etc/profile
/etc/profile.d/*.sh
Non-Login Shell Sessions
~/.bashrc
/etc/bash.bashrc
Testing Options
if [[ <-opt> xxx ]]; then
...
fi
Used frequently:
-e exist
-s NOT empty
-f regular file
-d directory
-S socket
-p named pipe
-h symbolic link
-r readable
-w writable
-nt newer than
-ot older than
-ef hard link
Loop Over Array
a=(1 2)
for i in ${a[*]}; do
echo $i
done